In the world of data structures and algorithms (DSA), stacks hold a significant position. Indeed, stacks are fundamental data structures that help solve numerous computational problems. For example: Python, being a versatile language, provides multiple ways to implement stacks efficiently. In this blog post, we will explore stacks in detail, including their definition, operations, implementation, and real-world applications.
Introduction
The term data structure (DSA) refers to data collection with well defined operations, behaviors, or properties. A stack is a linear structure where you implement operations in a LIFO (Last in First Out) manner, restricting insertions and deletions to occur only at the stack’s top.
LIFO means you will delete the element you inserted last first. The stack is also a dynamic data structure, as it can grow (an increase in the number of elements) or shrink (a decrease in the number of elements).
What is a Stack?
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. In simpler terms, the last element added to the stack is the first one to be removed. This property makes stacks particularly useful for scenarios that involve reverse order processing.
Why Use a Stack?
Stacks are simple yet powerful. They provide an intuitive way to manage data that follows a specific order. For instance, when you undo an action in a text editor, the application uses a stack to keep track of the changes. Similarly, stacks play a critical role in parsing expressions in compilers and managing the function call stack in programming languages.
Stack Operations
A stack supports the following primary operations:
- Push: Add an element to the top of the stack.
- Pop: Remove the top element from the stack.
- IsEmpty: Check whether the stack is empty.
- Peek (or Top): Retrieve the top element without removing it.
Rules in stacks
- Data can only be removed from the top (pop), i.e., the element at the top of the stack. The removal of an element from a stack is technically termed a POP operation.
- A new data element can only be added to the top of the stack (push). The insertion of elements in a stack is technically termed a PUSH operation.
Operations in Stack
push() operation in Stack
Push operation adds an item to the stack.
The figure below illustrates the PUSH operation in the stack:
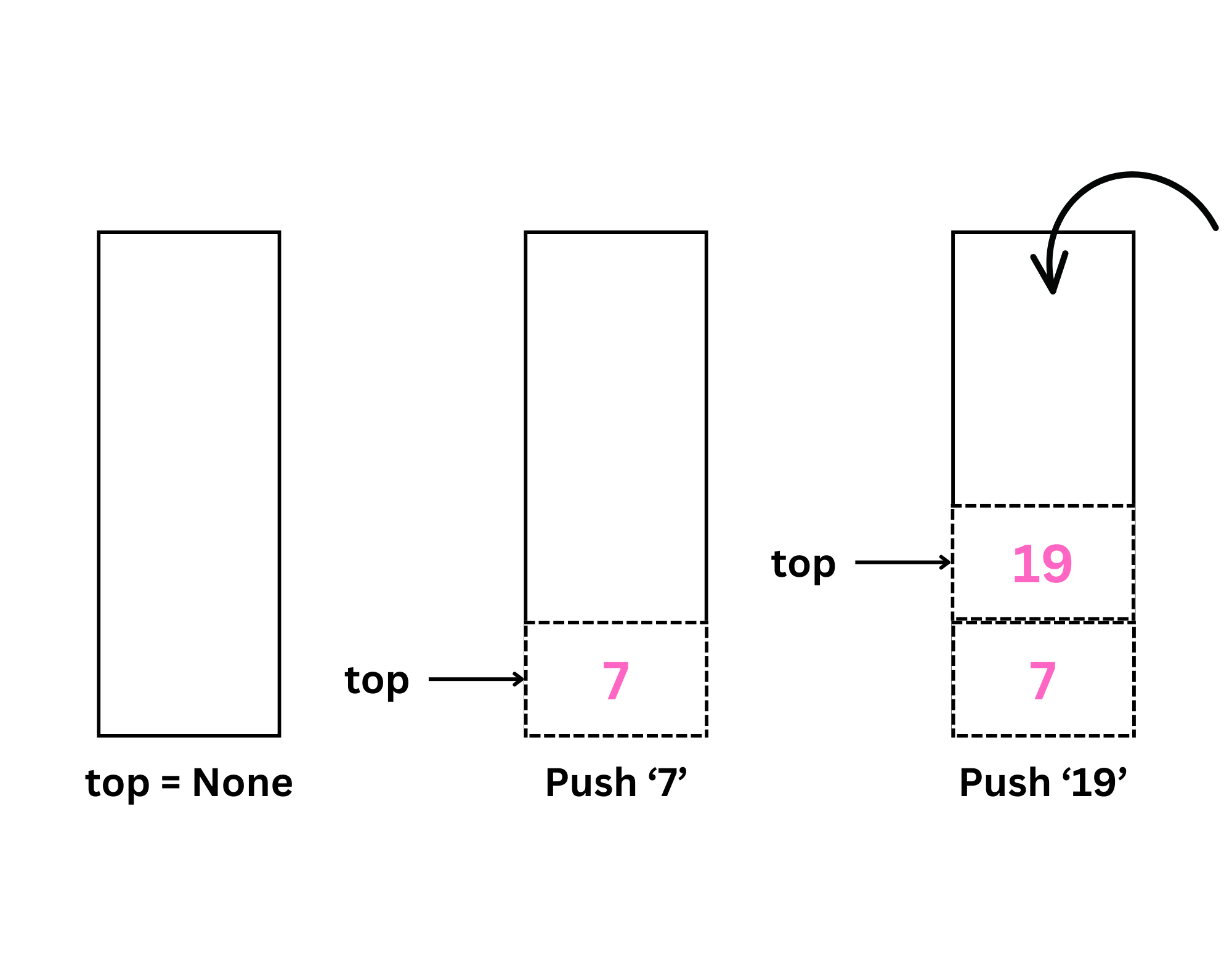
The ‘push()’ operation is one of the fundamental operations in the stack data structure. A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means that the last element added to the stack will be the first one to be removed.
Here’s a breakdown of the push() operation:
- Check if there is space available in the stack to add a new element. If the stack is full (in the case of a fixed-size stack), the operation may return an error or handle the overflow condition.
- If there is space, place the new element on top of the stack.
- Update the top pointer or index to reflect the addition of the new element.
For example in Python:
function push(stack, element):
if stack is full:
return "Stack Overflow"
else:
increment top index
stack[top] = element
Let’s consider a stack implemented using an array and perform a push() operation:
For example in Python:
# Python program to
# demonstrate stack implementation
# using list
stack = []
# append() function to push
# element in the stack
stack.append('x')
stack.append('y')
stack.append('z')
print('Initial stack')
print(stack)
# pop() function to pop
# element from stack in
# LIFO order
print('\nElements popped from stack:')
print(stack.pop())
print(stack.pop())
print(stack.pop())
print('\nStack after elements are popped:')
print(stack)
# uncommenting print(stack.pop())
# will cause an IndexError
# as the stack is now empty
For example in Python:
Initial stack
['x', 'y', 'z']
Elements popped from stack:
z
y
x
Stack after elements are popped:
[]
This demonstrates the basic ‘push()’ operation in a stack.
For example in Python:
function push(stack[], element):
if top == maxSize - 1:
print("Stack Overflow")
else:
top = top + 1
stack[top] = element
print(f"Element {element} pushed to stack")
// Example usage
stack = [None, None, None, None] // A stack with size 4
top = -1
push(stack, 10) // Output: Element 10 pushed to stack
push(stack, 20) // Output: Element 20 pushed to stack
Consider a stack implemented using an array. Here’s a detailed breakdown of the push() operation:
- Initialize the Stack: Suppose we have an array stack[] and an integer top that keeps track of the top position of the stack. Initially, top is set to -1, indicating an empty stack.
- Check for Overflow: Before adding an element, check if the stack is full. This can be done by comparing top with the maximum size of the stack minus one (e.g., if the stack size is maxSize, then check if top == maxSize – 1).
- Add the Element: If the stack is not full, increment the top by one (top = top + 1), and then place the new element at stack[top].
Edge Cases and Considerations
- Stack Overflow: If you attempt to push an element onto a full stack, it results in a stack overflow. This needs to be handled gracefully, usually by throwing an error or printing a message.
- Dynamic Stacks: In some implementations, stacks can dynamically resize when they become full, allowing for more flexible usage at the cost of additional complexity.
- Memory Management: Efficient memory management is crucial, especially in systems with limited resources. Proper handling of stack overflow and resizing ensures optimal performance.
Summary of push() in Stacks
The push() operation is essential for adding elements to a stack, following the LIFO principle. By checking for available space, inserting the new element, and updating the top pointer, the push() operation maintains the stack’s integrity. Understanding this operation lays the foundation for mastering stack-related algorithms and data structures.
pop() operation in Stack
Pop operation is used to remove an item from the stack.
The figure below illustrates the POP operation in the stack:
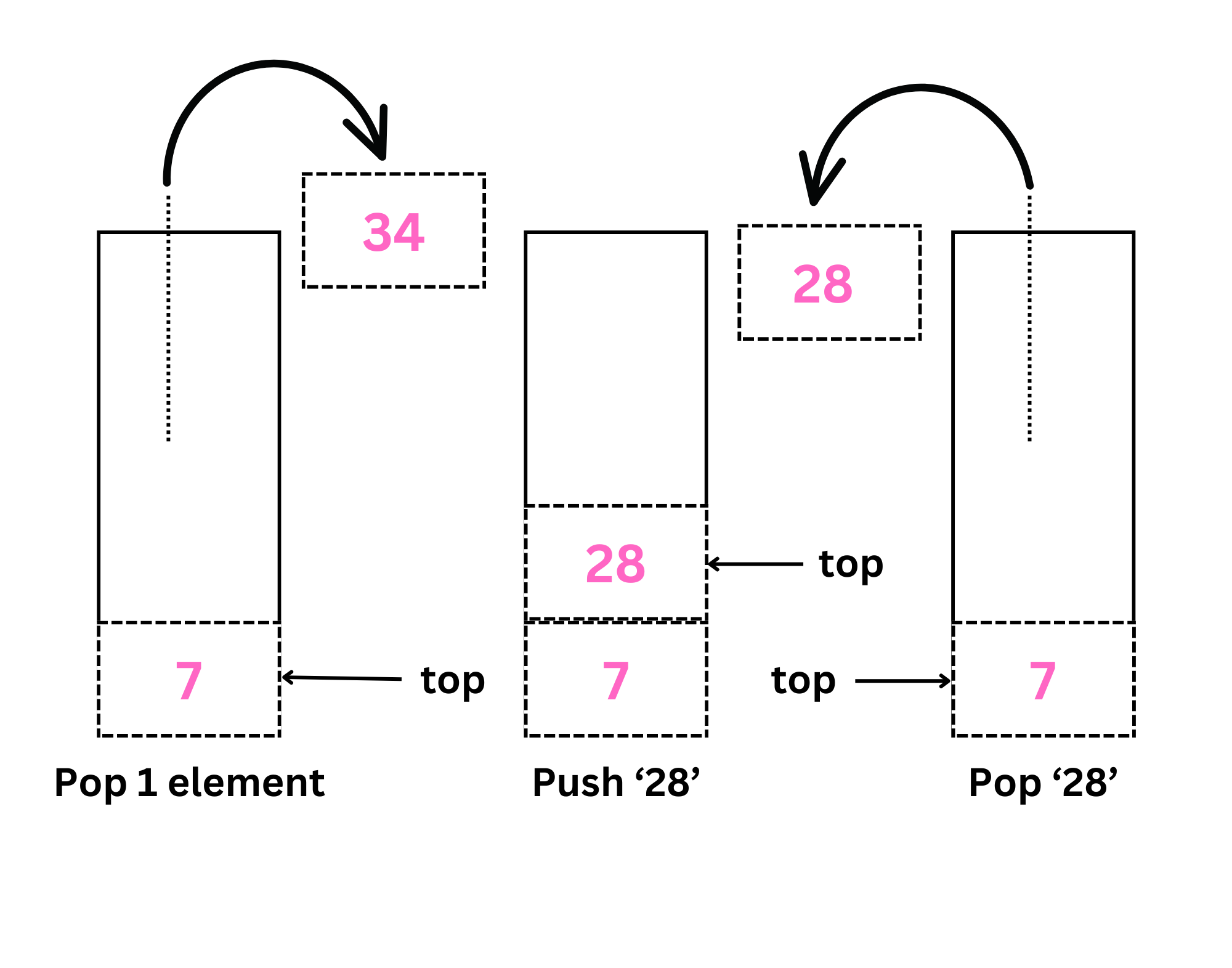
The ‘pop()’ operation is a fundamental action associated with the stack data structure. In essence, the ‘pop()’ operation removes the most recently added element from the stack, adhering to the Last In, First Out (LIFO) principle. Understanding how this operation works is crucial for anyone looking to master stack manipulation and algorithms that utilize this data structure.
How Does pop() Work?
When you perform a pop() operation on a stack, you are essentially doing two things:
Removing the Top Element: The pop() function removes the element that was most recently pushed onto the stack.
Returning the Removed Element: The function usually returns the value of the element that was removed, allowing you to use this value in further computations or logic.
Step-by-Step Execution of pop()
- Check if the Stack is Empty: Before performing the pop() operation, you must check if the stack is empty. Trying to pop an element from an empty stack typically raises an error or exception. This step ensures that the operation is safe and avoids potential runtime errors.
- Access the Top Element: Once you confirm that the stack is not empty, you access the top element. This element is the one that will be removed.
- Remove the Top Element: Next, you remove the element from the stack. In most implementations, this involves adjusting the pointer or index that keeps track of the top of the stack.
- Return the Top Element: Finally, the pop() operation returns the value of the removed element. This allows the calling function or method to use this value as needed.
Example 1:
Here is a simple implementation of the pop() operation in Python using a list to represent the stack:
#Simple Implementation of pop() in Python:
stack = []
stack.append(A) # This pushes A to the stack top
stack.append(1) # This pushes 1 to the stack top
stack.append(B) # This pushes B to the stack top
stack.append(2) # This pushes 2 to the stack top
stack.append(C) # This pushes C to the stack top
# printing the stack
while stack:
print(stack[-1], end=" ")
stack.pop()
# The above loop prints "C 2 B 1 A"
Benefits of Using pop()
- Efficiency: The pop() operation is typically very efficient, often executing in constant time O(1). This efficiency is due to the straightforward nature of the operation, which involves minimal steps.
- Simplicity: The operation is simple to implement and understand, making it a fundamental tool for developers working with stacks.
- Utility in Algorithms: The pop() operation is essential in many algorithms, such as depth-first search (DFS), expression evaluation (e.g., reversing Polish notation), and backtracking problems.
Transitioning Between Operations
While performing stack operations, it’s crucial to ensure smooth transitions between pushing and popping elements. Here are some tips:
- Always Check Stack State: Before popping, always check if the stack is empty to prevent errors.
- Use Auxiliary Functions: Functions like ‘is_empty()’ help manage transitions by providing a clean way to check the stack’s state.
- Handle Exceptions Gracefully: Implement error handling to manage cases where pop() is called on an empty stack.
By mastering the pop() operation and ensuring smooth transitions between stack operations, you can effectively leverage this data structure in various computational problems.
Edge Cases
When implementing the pop() operation for a stack in Python, there are several edge cases and considerations to keep in mind to ensure robustness and proper functionality. Here are some of the key points:
- Popping from an Empty Stack:
Ensure that the pop() method handles the situation where the stack is empty gracefully. This can be done by checking if the stack is empty before attempting to pop an element.
For example in Python:
def pop(self):
if not self.is_empty():
return self.items.pop()
else:
raise IndexError("pop from empty stack")
- Type Consistency:
Ensure that the stack only contains items of the expected type(s). While Python is dynamically typed, you might want to enforce type consistency for certain applications.
For example in Python:
def push(self, item):
if isinstance(item, (expected_type1, expected_type2)):
self.items.append(item)
else:
raise TypeError("Invalid item type")
Considerations
- Performance:
The pop() operation in a Python list is O(1) for popping from the end of the list, which is efficient. Ensure your stack implementation leverages this.
For example in Python:
def pop(self):
return self.items.pop() if not self.is_empty() else raise IndexError("pop from empty stack")
- Thread Safety:
If the stack is used in a multithreaded environment, consider thread safety. You might need to use locks or other synchronization mechanisms to ensure that operations on the stack are atomic.
Example in Python using ‘threading.Lock’:
import threading
class Stack:
def __init__(self):
self.items = []
self.lock = threading.Lock()
def is_empty(self):
return len(self.items) == 0
def push(self, item):
with self.lock:
self.items.append(item)
def pop(self):
with self.lock:
if not self.is_empty():
return self.items.pop()
else:
raise IndexError("pop from empty stack")
- Memory Management:
Be mindful of your memory usage. While Python’s garbage collector handles most memory management, holding references to objects in a stack can prevent them from being freed.
For example in Python:
def pop(self):
if not self.is_empty():
item = self.items.pop()
# Optional: Explicitly delete item if needed
del item
return item
else:
raise IndexError("pop from empty stack")
Here’s a complete example incorporating these considerations:
For example in Python:
import threading
class Stack:
def __init__(self):
self.items = []
self.lock = threading.Lock()
def is_empty(self):
return len(self.items) == 0
def push(self, item):
with self.lock:
self.items.append(item)
def pop(self):
with self.lock:
if not self.is_empty():
return self.items.pop()
else:
raise IndexError("pop from empty stack")
def peek(self):
if not self.is_empty():
return self.items[-1]
else:
raise IndexError("peek from empty stack")
def size(self):
return len(self.items)
# Example usage:
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.pop()) # Output: 2
print(stack.pop()) # Output: 1
try:
print(stack.pop()) # Raises IndexError: pop from empty stack
except IndexError as e:
print(e)
This example ensures that popping from an empty stack raises an appropriate exception, maintains thread safety, and provides a comprehensive stack implementation.
Summary of pop() in Stacks
The pop() operation in stacks removes the item from the top of the stack, following the Last-In-First-Out (LIFO) principle. To perform a pop, you first check if the stack is not empty (since you can’t pop from an empty stack). Then, you remove the top item and update the stack accordingly. For example, if a stack has items A, B, and C (with C on top), calling pop() will remove C, leaving A and B in the stack. This operation is crucial for tasks that require the most recently added data to be accessed or removed first.
isEmpty() operation in Stack
isEmpty operation is a boolean operation that is used to determine if the stack is empty or not.
What is the isEmpty() Operation?
The isEmpty() operation in a stack is a crucial method used to determine whether the stack contains any elements. Implementing and understanding this operation involves grasping the basics of stack data structure, which operates on the Last-In-First-Out (LIFO) principle.
Why is isEmpty() Important?
Knowing if a stack is empty is useful because it helps prevent errors when trying to remove an element from an empty stack. If we try to remove an element from an empty stack, we will encounter an error because there is nothing to remove. The isEmpty() method allows us to check first and avoid this problem.
Implementation of isEmpty()
To implement the isEmpty() operation, we need to consider the underlying structure of the stack. Typically, a stack can be implemented using an array or a linked list. The approach slightly varies depending on the chosen implementation.
Using an Array
When using an array to implement a stack, we maintain an index or counter that tracks the position of the top element. Here’s how we can implement the isEmpty() method:
- Initialize the Stack: We start by initializing the stack with a size and setting a top index to -1, indicating an empty stack.
- Check the Index: The isEmpty() method checks if the top index is -1. If it is, the stack is empty.
For example in Python:
class Stack {
private int[] stackArray;
private int top;
public Stack(int size) {
stackArray = new int[size];
top = -1;
}
public boolean isEmpty() {
return top == -1;
}
}
Using a Linked List
For a linked list implementation, we use nodes where each node points to the next element in the stack. The isEmpty() method checks if the reference to the top node is null.
- Define the Node Class: Create a node class to represent each element in the stack.
- Initialize the Stack: Set the top node to null to indicate an empty stack.
- Check the Top Node: The isEmpty() method checks if the top node is null.
For example in Python:
class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
class Stack {
private Node top;
public Stack() {
top = null;
}
public boolean isEmpty() {
return top == null;
}
}
Example in Pseudocode
Here is a simple pseudocode example to illustrate the isEmpty() method:
For example in Python:
class Stack:
def __init__(self):
self.items = [] # Initialize an empty list to store stack elements
def isEmpty(self):
if len(self.items) == 0: # Check if the list has no elements
return True # The stack is empty
else:
return False # The stack is not empty
# Usage
myStack = Stack() # Create a new stack
print(myStack.isEmpty()) # Output: True (because the stack is empty)
myStack.items.append(1) # Add an element to the stack
print(myStack.isEmpty()) # Output: False (because the stack is not empty)
Steps in Detail
- Initialization: We will start with an empty list self.items, that will store our stack elements.
- Check Length: In the isEmpty() method, we check the length of this list using len(self.items).
- Return Results: If the length is 0, we return True, indicating the stack is empty. Otherwise, we will return False.
Summary of isEmpty() in Stacks
The isEmpty() operation in a stack is a simple but crucial method. This helps ensure that we do not perform invalid operations on an empty stack. By checking if the stack is empty before removing elements, we can write safer and more reliable code. This method typically involves checking the top pointer or the length of the list, storing the stack elements, and returning a boolean value accordingly.
peek() or top() operation in Stack
peek or top operation is used to return the top element of the stack.
The peek() or top() operation in a stack allows you to look at the element on the top of the stack without removing it. This is useful when you need to know what the top element is but do not want to modify the stack.
How does Peek Work?
When you call the peek operation on a stack, it checks the top element and returns it. Here’s a step-by-step process for how it works:
1. Check if Stack is Empty:
- First, the peek operation checks if the stack is empty. If the stack is empty, there’s no top item to look at, so the operation might return an error or a special value indicating the stack is empty.
2. Access to the top item:
- If the stack is not empty, the operation accesses the item at the top of the stack.
3. Return the top item:
- Finally, the peek operation returns the top item without removing it from the stack.
Why Use peek()?
- Preview Top Item: Peek is useful when you want to know what’s on top of the stack without modifying the stack. For example, if you’re working with a stack of tasks, you might want to see the next task to do without starting it.
- Safety Inspection: It lets you inspect the stack’s top elements safely, ensuring that the element remains in place for future operations.
- Making Decisions: You might need to decide what to do next based on the top item. For instance, in some algorithms, you need to check the top item to decide whether to add more items or remove some.
An Example in Simple Terms
Let’s say you have a stack of books. The stack currently looks like this:
- Top: “Book 3”
- Middle: “Book 2”
- Bottom: “Book 1”
When you perform a peek() operation:
- Look at the Top book: You see “Book 3”.
- Don’t Remove It: You don’t take “Book 3” off the stack, you just look at it.
- Result: The peek() operation tells you the top book is “Book 3”.
For example in Python:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
else:
return None
def is_empty(self):
return len(self.items) == 0
# Create a stack and add some items
my_stack = Stack()
my_stack.push("Book 1")
my_stack.push("Book 2")
my_stack.push("Book 3")
# Use peek() to see the top item
top_item = my_stack.peek()
print(top_item) # Output: Book 3
Summary of peek() in Stacks
The peek() operation in a stack is a handy way to view the top element without removing it. This ensures that the stack remains unchanged while providing the necessary information about the top element. This operation is especially useful when you need to make decisions based on the top element but do not want to alter the stack’s state.
Summary of Stacks
Understanding Stacks: Push, Pop, and Peek Operations
A stack is a basic data structure in computer science. Think of it like a stack of plates: you can only add or remove plates from the top. Stacks follow the Last-In-First-Out (LIFO) principle, meaning the last item you put on the stack is the first one you take off. Let’s break down the three main operations you can perform on a stack: push, pop, and peek.
Push Operation
The push operation adds an item to the top of the stack. Imagine you’re stacking plates. When you add a plate, you place it on top of the stack.
Here’s how it works:
- Identify the item you want to add to the stack.
- Place the item on top of the stack.
- Update the stack to reflect the new item on top.
For example, if your stack already has plates labeled A, B, and C (with C on top), and you push plate D, your stack will now have plates A, B, C, and D (with D on top).
Pop Operation
The pop operation removes the item from the top of the stack. Continuing with our plate analogy, when you take a plate off, you remove the one on the very top.
Here’s the process:
- Check if the stack is not empty (you can’t pop from an empty stack).
- Remove the top item from the stack.
- Update the stack to reflect the removal.
For instance, if your stack has plates A, B, C, and D (with D on top), and you pop an item, D will be removed, leaving plates A, B, and C (with C now on top).
isEmpty Operation
The isEmpty operation checks whether the stack is empty. This is like checking if there are any plates in the stack.
Here’s how it works:
- Check the stack to see if it has any items.
- Return a boolean value: true if the stack is empty, false if it has items.
For example, if your stack has plates A, B, and C, isEmpty will return false. If there are no plates, isEmpty will return true.
Peek Operation
The peek operation allows you to see the item on the top of the stack without removing it. This is like looking at the top plate on the stack without picking it up.
Here’s what you do:
- Check if the stack is not empty (you can’t peek at an empty stack).
- Look at the top item and note what it is.
For example, if your stack has plates A, B, C, and D (with D on top), a peek operation will let you see D without removing it.
Using Stacks in Programming
In programming, stacks are useful for various tasks like managing function calls, undo mechanisms in text editors, and more.
Summary of Key Points:
- Push: Adds an item to the top of the stack.
- Pop: Removes the top item from the stack.
- isEmpty: Checks if the stack is empty.
- Peek: Shows the top item without removing it.
Stacks are simple yet powerful tools in computing, and mastering these basic operations will help you understand more complex data structures and algorithms.
Discover more from lounge coder
Subscribe to get the latest posts sent to your email.