In the world of data structures, the doubly linked list stands out as a versatile and powerful tool. Unlike its simpler counterpart, the singly linked list, a doubly linked list allows traversal in both directions. This unique capability makes it an excellent choice for various applications where bidirectional navigation is essential.
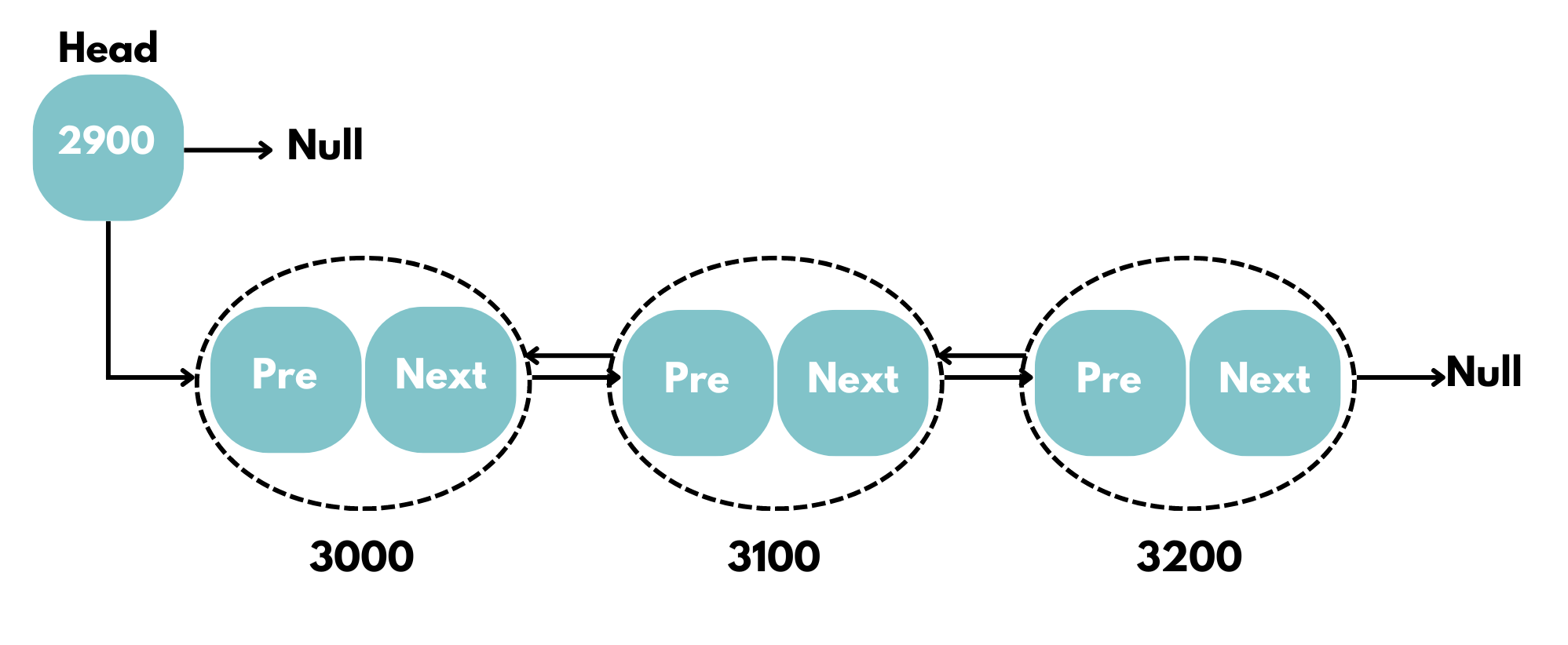
What is a Doubly Linked List?
A doubly linked list consists of nodes. Each node contains three components: the data, a pointer to the next node, and a pointer to the previous node.
- Data: The value or information stored in the node.
- Next Pointer: A reference to the next node in the sequence.
- Previous Pointer: A reference to the previous node in the sequence.
By having pointers to both the next and previous nodes, the doubly linked list enables seamless traversal in both forward and backward directions. This structure contrasts with a singly linked list, where each node only points to the next node.
Key Components of a Doubly Linked List
- Nodes: The building blocks of a doubly linked list. Each node holds data and two pointers: one for the next node and one for the previous node.
- Head: The first node in the list. Its previous pointer is null.
- Tail: The last node in the list. Its next pointer is null.
Operations on a Doubly Linked List
To fully appreciate the doubly linked list, let’s delve into its various operations:
Insertion:
- At the Beginning: To insert a node at the beginning, you create a new node, set its next pointer to the current head, and update the head’s previous pointer to this new node. Finally, update the head to this new node.
- At the End: To insert at the end, create a new node, set its previous pointer to the current tail, and update the tail’s next pointer to this new node. Then, update the tail to this new node.
- In the Middle: To insert a node in the middle, update the pointers of the adjacent nodes to point to the new node, ensuring correct assignment of both the next and previous pointers.
Deletion:
- From the Beginning: To delete the head node, update the head to the next node and set the new head’s previous pointer to null.
- From the End: For deleting the tail, update the tail to the previous node and set the new tail’s next pointer to null.
- From the Middle: For middle deletions, update the pointers of the adjacent nodes to bypass the node you are deleting.
Traversal:
- Forward Traversal: Start from the head and follow the next pointers until you reach the tail.
- Backward Traversal: Begin at the tail and follow the previous pointers back to the head.
Advantages of Linked Lists
Linked lists are a fundamental data structure in computer science, offering several benefits over other structures like arrays. Here are the key advantages:
Dynamic Size
- Flexible Memory Allocation: Linked lists allocate memory as needed. Unlike arrays, which require a predefined size, linked lists can grow or shrink dynamically, making them suitable for applications where the number of elements is unpredictable.
Efficient Insertions and Deletions
- O(1) Insertions and Deletions: Adding or removing elements from a linked list is efficient. Unlike arrays, where shifting elements is necessary, linked lists only require pointer updates, making these operations quick and consistent in performance.
No Wasted Memory
- Memory Utilization: Linked lists allocate memory exactly as needed. This means there is no wasted space due to preallocation or resizing issues common with arrays.
Ease of Implementation of Certain Data Structures
- Foundation for Other Data Structures: Linked lists serve as the foundation for more complex data structures like stacks, queues, and graph adjacency lists. Moreover, their flexibility and ease of use make them ideal for these implementations.
Simplified Memory Management
- No Need for Contiguous Memory: Unlike arrays, which require a block of contiguous memory, linked lists can be scattered throughout memory. This feature makes it easier to allocate and manage memory without worrying about fragmentation.
Bidirectional Traversal (in Doubly Linked Lists)
- Enhanced Traversal Capabilities: In a doubly linked list, you can traverse both forward and backward. This bidirectional capability is particularly useful in certain applications, like navigation systems and undo-redo functionalities.
Reduced Complexity for Certain Operations
- No Shifting of Elements: When inserting or deleting elements, linked lists do not require shifting elements as arrays do. This lack of shifting simplifies operations and reduces the computational overhead.
Facilitation of Recursive Algorithms
- Natural Fit for Recursion: Linked lists are inherently recursive data structures; therefore, they are a natural fit for recursive algorithms. Since each node points to the next, they provide a straightforward way to implement recursive solutions.
Disadvantages of Doubly Linked Lists
While doubly linked lists offer numerous advantages, they also come with certain drawbacks. Understanding these disadvantages is crucial when deciding whether to use this data structure for a particular application.
Increased Memory Usage
Each node in a doubly linked list contains an additional pointer compared to a singly linked list. This means that:
- Memory Overhead: The extra memory required to store the previous pointer can be significant, especially in memory-constrained environments or when dealing with large datasets.
More Complex Implementation
Managing a doubly linked list is more complex than managing a singly linked list because:
- Pointer Management: Each operation (insertion, deletion, traversal) requires careful updating of both next and previous pointers, increasing the potential for errors.
- Additional Code: More complex operations result in more extensive and intricate code, which can be harder to debug and maintain.
Slower Operations
For certain operations, the additional complexity of maintaining two pointers can lead to:
- Insertion and Deletion Overhead: Although doubly linked lists allow for more flexible insertion and deletion, each operation typically involves updating multiple pointers, which can be slower than the corresponding operations in singly linked lists, especially when considering the overhead of ensuring all pointers are correctly updated.
Potential for Increased Complexity
With the additional flexibility comes the potential for more complicated algorithms:
- Algorithm Complexity: Algorithms that operate on doubly linked lists may need to account for the additional pointers, leading to more complex logic and increased likelihood of bugs.
Risk of Data Corruption
If pointers are not managed correctly, the risk of data corruption increases:
- Pointer Errors: Incorrectly updating the next or previous pointers can lead to broken links in the list, making the data structure unusable and potentially causing application crashes or unpredictable behavior.
Applications of Doubly Linked Lists
Doubly linked lists are used in various applications, including:
- Navigation Systems: Doubly linked lists can efficiently manage back and forth navigation, such as in web browsers where the user can move forward and backward through pages.
- Undo/Redo Functionality: In software applications, doubly linked lists can track changes and enable the undo and redo functionalities.
- Music Playlists: They allow users to navigate between songs in both directions seamlessly.
Summary
- In summary, the doubly linked list is a dynamic and adaptable data structure that provides significant advantages over the singly linked list. Its ability to facilitate bidirectional traversal, ease of deletion, and versatility in operations make it a valuable tool in various applications.
- Understanding how to implement and utilize a doubly linked list can significantly enhance your programming and problem-solving skills. So, next time you need a data structure that offers both flexibility and efficiency, consider leveraging the power of the doubly linked list.
Discover more from lounge coder
Subscribe to get the latest posts sent to your email.