What is a Singly Linked List?
A singly linked list is the sort of linked list in which each node has just one link, which leads to the next node in the list.
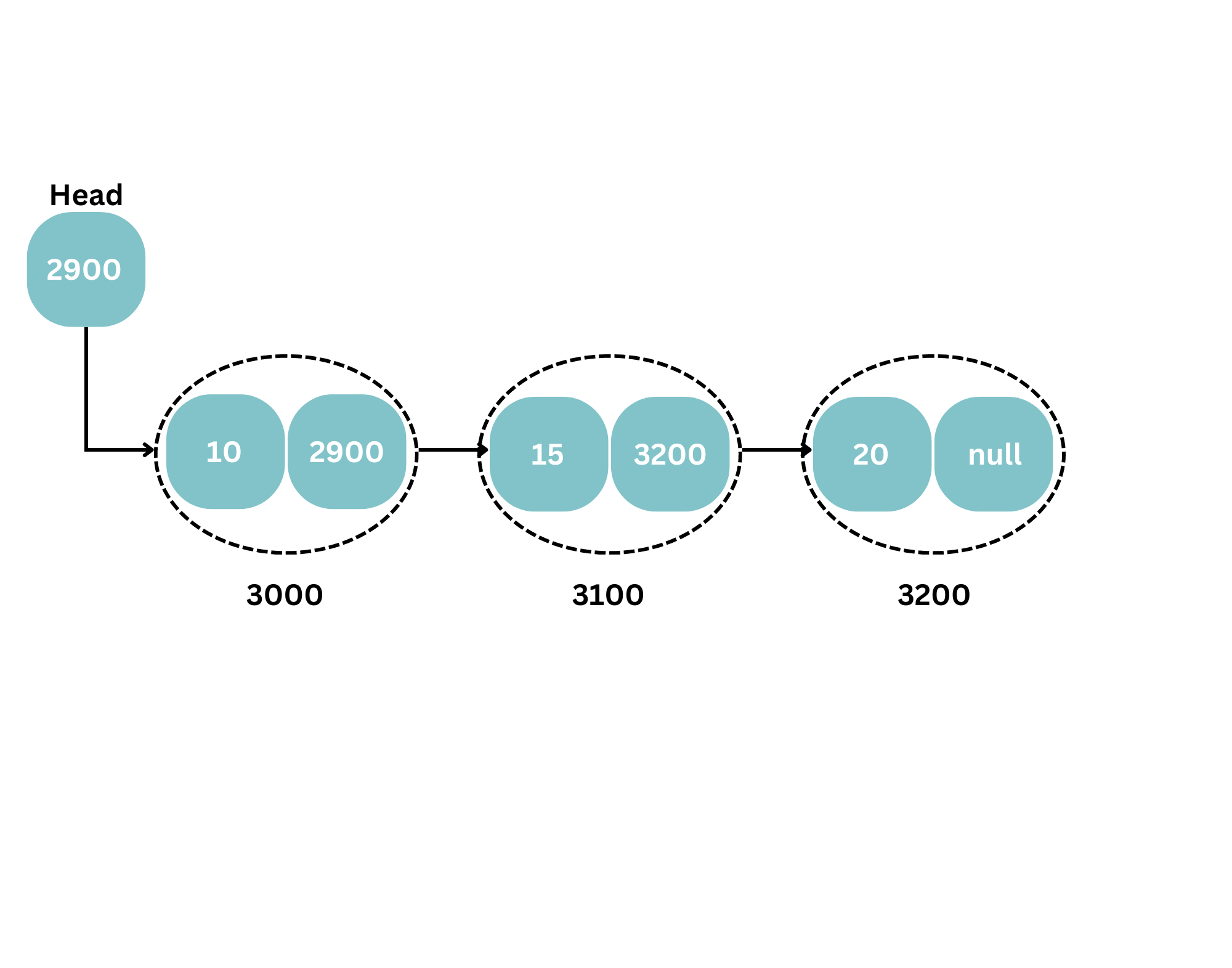
- A singly linked list is a type of linear data structure where each element, called a node, contains two parts: data and a reference (or link) to the next node in the sequence.
- Unlike arrays, the nodes in a singly linked list are not stored in contiguous memory locations, allowing for dynamic memory allocation and efficient insertions and deletions.
Structure of a Singly Linked List
Each node in a singly linked list consists of:
- Data: The value or information stored in the node.
- Next: A pointer that references the next node in the list. If the node is the last one, the next pointer points to null, indicating the end of the list.
Here’s a simple representation of a singly linked list:
[Data | Next] -> [Data | Next] -> [Data | Next] -> null
Basic Operations
Singly linked lists support several fundamental operations, making them versatile for various applications. Let’s examine some of these operations:
Insertion
- At the Beginning: To insert a new node at the start, you create a new node and set its next pointer to the current head of the list. Then update the head to this new node.
- At the end: To insert at the end, traverse the list until you reach the last node. Set the next pointer of this node to the new node.
- At a Given Position: To insert at a specific position, traverse the list to the desired point, update the next pointer of the preceding node, and link the new node to the subsequent node.
Deletion
- From the Beginning: To delete the first node, update the head to point to the next node.
- From the End: To delete the last node, traverse the list until you reach the second-to-last node, then set its next pointer to null.
- From a Given Position: To delete a node from a specific position, traverse the list to the node just before the one you want to delete. Update its next pointer to skip the node to be deleted.
Traversal
- To traverse a singly linked list, start from the head and move through each node by following the next pointers until you reach the end (null).
Search
- To search for an element, start from the head and traverse through the list, comparing each node’s data with the target value.
Characteristics of a Singly Linked List
Singly linked lists are a fundamental data structure in computer science, distinguished by several unique characteristics that make them suitable for various applications. Here’s a detailed look at these characteristics:
Node-Based Structure
A singly linked list is composed of nodes. Each node contains:
- Data: The value or information stored in the node.
- Next Pointer: A reference to the next node in the sequence. The last node’s next pointer points to null, indicating the end of the list.
Linear Order
- The nodes in a singly linked list are arranged in a linear order. Each node points to exactly one successor, creating a straight, unidirectional chain from the head to the end (null).
Dynamic Size
- Unlike arrays, which have a fixed size, singly linked lists are dynamic. They can grow and shrink in size as nodes are added or removed. This flexibility allows for efficient memory usage, as nodes are allocated only as needed.
Efficient Insertions and Deletions
Insertions and deletions in a singly linked list are efficient, particularly when operations are performed at the beginning or middle of the list. These operations typically involve updating a few pointers, rather than shifting elements as required in arrays.
- Insertion at the Beginning: Requires updating the next pointer of the new node and the head pointer.
- Deletion at the Beginning: Involves updating the head pointer to the next node.
Sequential Access
- Access to elements in a singly linked list is sequential. To retrieve a specific element, you must traverse the list from the head node, following the next pointers until you reach the desired node. This characteristic makes random access less efficient compared to arrays.
No Backward Traversal
- Singly linked lists do not support backward traversal. Since each node points only to its successor, you cannot move backwards through the list. For operations requiring reverse traversal, a doubly linked list or another data structure might be more suitable.
Memory Usage
- Each node in a singly linked list requires extra memory for storing the next pointer, in addition to the data. While this overhead is generally minimal, it is a consideration in memory-constrained environments.
Simplicity
- The structure of singly linked lists is simple and easy to implement. This simplicity makes them a popular choice for implementing basic data structures such as stacks, queues, and simple associative arrays.
No Fixed Memory Allocation
- Nodes in a singly linked list are not stored in contiguous memory locations. Each node is allocated memory independently, allowing the list to utilize fragmented memory effectively.
Ease of Implementation
- Singly linked lists are straightforward to implement. Basic operations such as insertion, deletion, and traversal can be easily coded, making them a good starting point for understanding more complex data structures.
Advantages of Singly Linked Lists
Singly linked lists offer several benefits:
- Dynamic Size: Unlike arrays, linked lists do not have a fixed size. You can add or remove elements without worrying about memory limitations.
- Ease of Insertion/Deletion: Inserting or deleting elements is more efficient than in arrays, especially at the beginning or middle of the list, as it only involves updating pointers.
- Efficient Memory Utilization: Since nodes are allocated memory as needed, there is no wasted space, unlike arrays that may allocate extra space.
Disadvantages of Linked Lists
While singly linked lists offer several benefits, they also come with a set of disadvantages. Understanding these drawbacks is crucial for making informed decisions about when to use this data structure. Here’s a detailed look at the disadvantages of singly linked lists:
Sequential Access
- One of the primary drawbacks of singly linked lists is that they support only sequential access. To access a specific element, you must start at the head node and traverse the list until you reach the desired node. This makes operations like searching for an element or accessing an element at a particular index less efficient compared to arrays, which allow direct access via indexing.
Increased Memory Usage
- Each node in a singly linked list requires extra memory for storing the next pointer. In memory-constrained environments, this overhead can be significant, especially if the list contains a large number of nodes.
No Reverse Traversal
- Singly linked lists do not allow traversal in the reverse direction. Each node points only to its successor, so moving backwards through the list is not possible. This limitation makes certain algorithms and operations more complex or inefficient, particularly those that require bidirectional traversal.
Complexity in Insertion/Deletion in Specific Positions
- While insertions and deletions at the beginning of the list are straightforward, performing these operations at specific positions requires traversal to that position, which can be time-consuming. For instance, to delete a node in the middle of the list, you need to traverse the list to find the preceding node and update its next pointer, which takes O(n) time in the worst case.
Poor Cache Performance
- Due to the non-contiguous memory allocation of nodes, singly linked lists often exhibit poor cache performance. Modern CPUs cache contiguous memory locations for faster access. Since linked list nodes are scattered throughout memory, accessing them can result in more cache misses compared to arrays, which are stored in contiguous memory blocks.
Extra Space for Pointers
- In addition to the data, each node in a singly linked list stores a pointer to the next node. This extra space can add up, particularly in large lists, leading to inefficient use of memory. Arrays, on the other hand, do not have this overhead since they do not store pointers.
Difficulty in Debugging and Error Handling
- Debugging issues in singly linked lists can be challenging. Problems such as incorrect pointer assignments can lead to memory leaks, segmentation faults, or infinite loops. Detecting and fixing these issues requires careful inspection of pointer operations, making debugging more complex compared to simpler data structures like arrays.
Limited Functionality Compared to Doubly Linked Lists
- Singly linked lists offer fewer features compared to doubly linked lists. For instance, singly linked lists cannot efficiently implement certain operations that require reverse traversal or access to previous nodes. In cases where such functionality is needed, doubly linked lists, which maintain pointers to both the next and previous nodes, are more suitable.
Summary
While singly linked lists are useful and versatile, they come with several disadvantages that can impact their performance and usability in certain scenarios. Sequential access, increased memory usage, lack of reverse traversal, and poor cache performance are some of the key drawbacks. Understanding these limitations helps in choosing the appropriate data structure for specific applications, ensuring efficient and effective program design.
Discover more from lounge coder
Subscribe to get the latest posts sent to your email.