What is a Linked List?
A linked list is a versatile and dynamic data structure, fundamental in computer science. Unlike arrays, linked lists do not store their elements in contiguous memory locations. Instead, each element, known as a node, consists of two parts: the data and a reference (or link) to the next node in the sequence. This unique structure enables linked lists to manage memory efficiently, making them highly suitable for various applications.
Structure of a Linked List
A linked list is composed of nodes, where each node contains two main components: the data and the reference (or link) to the next node. This structure allows linked lists to store a sequence of elements in a non-contiguous memory fashion.
Basic Structure of a Node
A typical node in a linked list has the following structure:
- Data Part: This part stores the actual value or information the node holds.
- Link Part: This part is a reference (or pointer) to the next node in the sequence.
Here’s a simplified visual representation of the node in a single linked list:
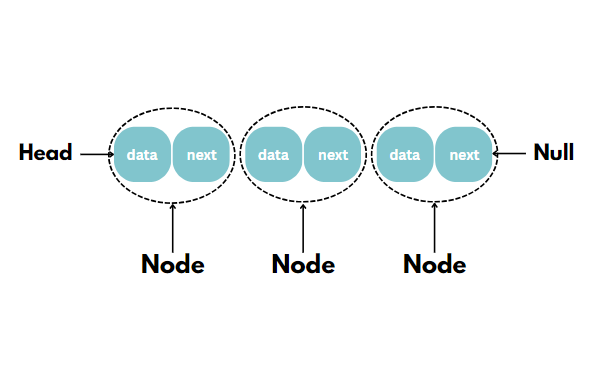
[ Data | Next ]
In a more formal notation, if we define a node class in a programming language like Python, it might look like this:
class Node:
def __init__(self, data=None):
self.data = data # The data part of the node
self.next = None # The link to the next node
Key Characteristics of Linked Lists
Dynamic Size
Linked lists have the remarkable ability to grow and shrink dynamically. This flexibility stems from their non-contiguous memory allocation. As a result, you can add or remove nodes without worrying about resizing operations, which are often necessary with arrays. When a node is added or removed, only the links between nodes need updating, making the process efficient and straightforward.
Ease of Insertion and Deletion
One of the most compelling advantages of linked lists is the ease of insertion and deletion. In an array, inserting or deleting an element requires shifting other elements to maintain the sequence. However, in a linked list, you only need to update the pointers. Here’s a closer look at these operations:
Insertion
- At the Beginning: To insert a new node at the start, you simply create a new node and adjust its link to point to the current first node. Then, update the head pointer to this new node.
- At the End: For adding a node at the end, traverse the list to find the last node, create a new node, and set the last node’s link to this new node.
- In the Middle: When inserting in the middle, traverse the list to the desired position, adjust the links of the surrounding nodes to include the new node, and you’re done.
Deletion
- At the Beginning: To delete the first node, simply update the head pointer to the second node.
- At the End: For removing the last node, traverse the list to find the second-to-last node, then set its link to null.
- In the Middle: Deleting a node from the middle involves updating the link of the preceding node to point to the node following the one being removed.
These operations showcase the efficiency of linked lists in managing dynamic datasets.
Advantages and Use Cases
Linked lists offer several benefits over other data structures. Their dynamic size and efficient insertion and deletion operations make them ideal for applications where the dataset frequently changes. Additionally, they are particularly useful for implementing other data structures like stacks, queues, and graphs.
Advantages:
- Memory Efficiency: Linked lists use memory proportional to the number of elements, avoiding the need for pre-allocating memory as with arrays.
- Flexibility: They easily accommodate varying sizes, which is beneficial in situations where the number of elements is unpredictable.
- Efficient Insertions/Deletions: As previously mentioned, updating pointers is quick and easy, enhancing performance for these operations.
Use Cases:
- Dynamic Memory Allocation: Linked lists excel in scenarios requiring frequent memory allocation and deallocation.
- Implementing Other Data Structures: They serve as the foundation for more complex structures such as stacks, queues, and adjacency lists for graphs.
- Undo Mechanisms: Applications with undo features often use linked lists to keep track of actions, allowing for easy traversal and modification.
Summary
In conclusion, linked lists are a fundamental data structure with dynamic size, efficient insertion and deletion capabilities. Their unique structure and advantages make them indispensable in various applications, particularly where memory efficiency and flexible operations are paramount. Understanding and leveraging linked lists can significantly enhance your ability to manage and manipulate dynamic datasets effectively.